An open-source SDK for integrating in-app purchases on React Native
Implement in-app purchases in 30 minutes.
Out-of-the-box back end for React Native in-app purchases
Why choose Adapty SDK?
Correct subscription state at any moment
Rest assured you’ll always get the correct subscriber state across all platforms.
Server-side receipt validation
No need to worry about the correctness and safety of purchase validation.
Handling all kinds of subscription states
Free trials, upgrades, promo offers, family sharing, renewals, and more.
Enterprise-ready platform with a short release cycle
>99.99% SLA reliability and regular product updates.
Configuring platforms
Installing Adapty SDK
Adapty.activate(
"PUBLIC_SDK_KEY",
customerUserId: "YOUR_USER_ID"
)
"PUBLIC_SDK_KEY",
customerUserId: "YOUR_USER_ID"
)
Processing purchasing events
Fast and easy integration
Spend only a couple of hours to integrate the Adapty SDK into your iOS app and we’ll take care of the rest.
Just 5 SDK methods to implement React Native in-app purchases
// Your app's code
try {
const profile = await adapty.makePurchase(product);
const isSubscribed = profile?.accessLevels['YOUR_ACCESS_LEVEL']?.isActive;
if (isSubscribed) {
// grant access to features in accordance with access level
}
} catch (error) {
// handle the error
}
// Your app's code
try {
const profile = await adapty.restorePurchases();
const isSubscribed = profile.accessLevels['YOUR_ACCESS_LEVEL']?.isActive;
if (isSubscribed) {
// restore access
}
} catch (error) {
// handle the error
}
// Your app's code
try {
await adapty.identify("YOUR_USER_ID");
// successfully identified
} catch (error) {
// handle the error
}
 
 
 
// Your app's code
try {
await adapty.updateProfile(params);
} catch (error) {
// handle `AdaptyError`
}
import { adapty, AttributionSource } from 'react-native-adapty';
import appsFlyer from 'react-native-appsflyer';
appsFlyer.onInstallConversionData(installData => {
try {
// It's important to include the network user ID
const networkUserId = appsFlyer.getAppsFlyerUID();
adapty.updateAttribution(installData, AttributionSource.AppsFlyer, networkUserId);
} catch (error) {
// handle error
}
});
// ...
appsFlyer.initSdk(/*...*/);
People from all kinds of businesses turn to Adapty to grow their revenue
What do you get with Adapty?
Adapty SDK provides tremendous possibilities for growing app revenue
Real-time analytics for your React Native App
Rely on the data with 99.5% accuracy with App Store Connect.
Get startedGravity Fit
Health & Fitness
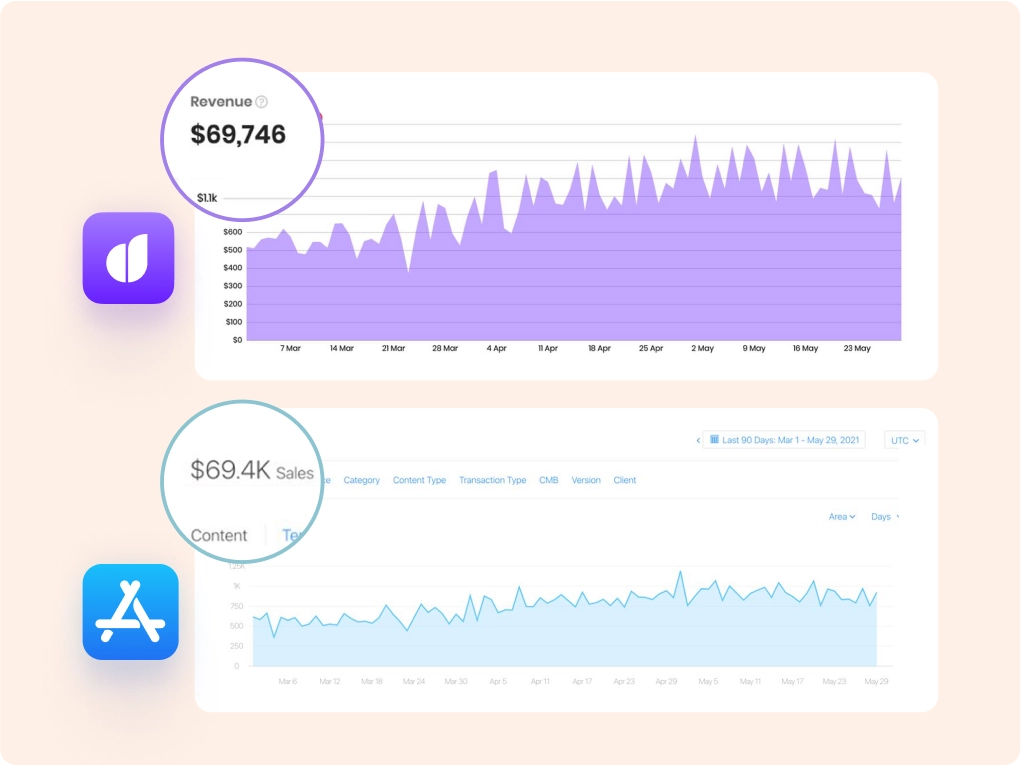
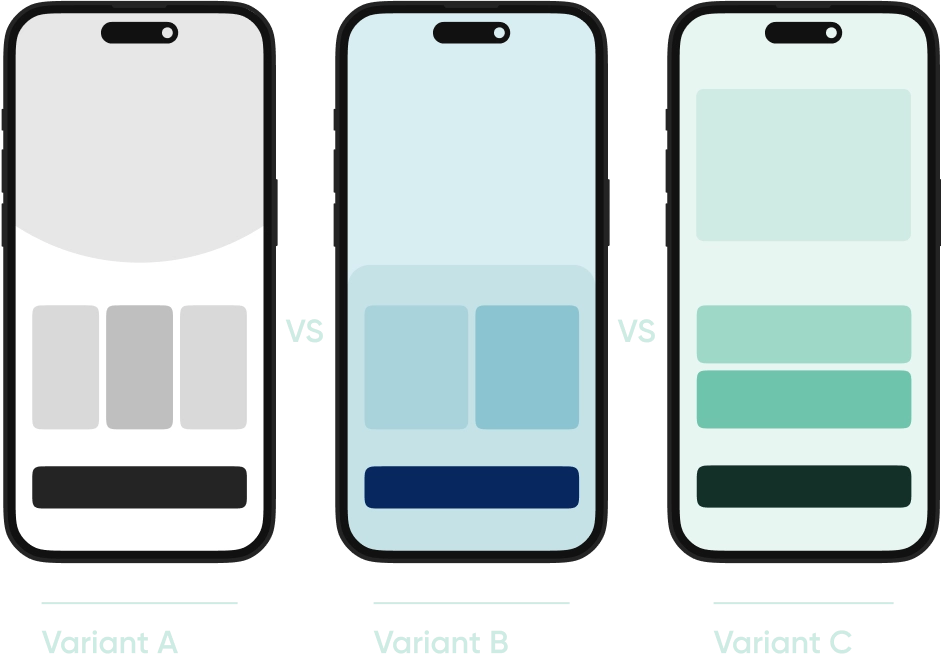
Paywall A/B testing
Find the most profitable paywall and grow your app’s revenue.
Explore A/B testingMentalGrowth
Health & Wellness app
Remote config for paywalls
Change your paywall remotely without having
to re-release the app.
Try it now
Smitten - Dating
Lifestyle
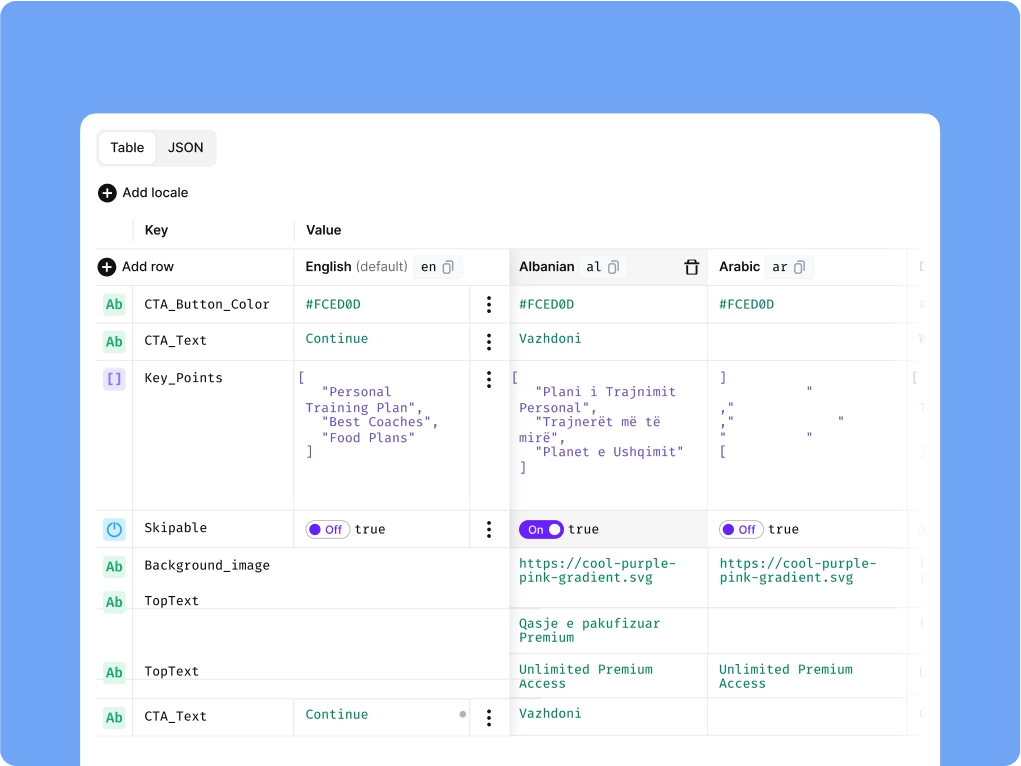